When developers first start their journey, sometimes syntax is easy. You’ve memorized a few commands, classes, and method names, and you can make simple applications quite easily. Also, there is plenty of docs and tutorials online - as well as Stack Overflow - surely copying-and-pasting your way through would be enough, right?
Let’s try to answer the question: how to start approaching problem solving for any given task?
Well, the real problem most actually face on a day-to-day basis when trying to program is not determining which lines of ifs and for loops go where. The problem starts at… well, the problem. Where to start? How to approach a solution to a given task or problem you are facing?
Some people, when confronted with a problem, think “I know, I’ll use regular expressions.” Now they have two problems.
~ Jamie Zawinski
Learning to put theory to practice
In my time developing I tried hard to learn to recognize the most important skills a developer has in their tool set - and one of the biggest hurdles for starting developers - is the ability to know how to face any problem - at least, in the context of development.
When an up-coming developer gets a new task – either from someone else (a boss, a colleague) or that they themselves define (for challenge, for proof of concept, for learning) - they often get stuck at the first hurdle - “where do I start?”
Now that you can create simple sentences – you have no idea where to begin when trying to write entire paragraphs or essays. How does it my “hello world” actually do what it should? How do I take what I know and combine the parts so they work with each other?
Here, I will try to give you some thought experiments and tools so you can be self-sufficient when it comes to starting a new coding task, whether it is adding to existing code or beginning from scratch.
We will start very simple, and then try to apply the same ideas to more complex tasks later on. So while it may seem very stupid and superficial at first, it will come together soon enough.
Defining a “problem”
A problem in our context is any task that has been given, however large or small.
For example, here are some small task examples:
- Add a “Contact” button to the home page that links to the contact page [design attached]
- Create a program that calculates the area of a rectangle
And here are some larger tasks:
- Create a snake game
- Create a to-do list application
All tasks are completely unique, and yet somewhat alike. How do we approach each of these from a development perspective?
The methodology - The 5 Steps of Completion
Let’s start introducing our frame of thought. We need to define the context of our task, so we can ask the proper questions right after.
1. Ask Questions
Let’s take a basic example to start.
Given story: Add a “Contact” button to the home page that links to the contact page
Ask yourself the following questions:
-
What is the current state? (i.e., from what point do I start?)
This won’t become a task later, but will be important for you to picture a “delta” of sorts, which draws a path in your mind from A (what there is) to B (what you want achieve). -
What does the user expect to see change?
-
What interactions can the user make with the change?
2. Give Answers
Let’s attempt to answer these questions for this case, one by one.
-
Q: What is the current state?
A: The user can see a website, with various sections, images and links. In the home page, there is no button that links to the “Contact” page.
-
Q: What does the user expect to see change?
A: The user should see a new button with the text “Contact”
-
Q: What interactions can the user make with the change?
A: The user should be able to click the button. Clicking it will point to the “Contact” page.
We didn’t learn a lot of new information by breaking down these questions. But we did gain separation which is critical. Now we have a list of tasks, instead of one task.
Why is that helpful?
3. Break it down
Large and small tasks alike – even the smallest of addition to your code, can be broken down even further. It is crucial that we learn to break things down as developers, because then we can create actionable items which we can start working on.
The example above might be a bit trivial, but it gets the point across - we can break everything down further, into infinity. Really, this has no limit. But we should stop somewhere…
So what next?
4. Create Actionables
Now what we want to do is take the answers from above, and compile them into a list of actions. The first question is redundant as it describes what is going on right now. So we will list the rest and try to create action items from them:
- The user should see a new button with the text “Contact”
- Show a button on the page
- Have the text say “Contact”
- Design the button to fit with the design
- The user should be able to click the button. Clicking it will point to the “Contact” page.
- Attach a click “handler” to the button
- Make it redirect the page to the desired URL
We are making some progress. We now have a list of tasks to work with. See how 2 small questions already turned into 5 tasks, and that is for a tiny problem.
5. Solve It
Now that we have tasks - we can get crackin’.
For the sake of this let’s assume we are developers that are relatively new to web development. What would we do to approach this task?
When in doubt, look it up
Google, Duck Duck Go, Brave, whatever. What do you choose? Your preference. What would we search for here exactly?
The trick is to be as broad as possible, and when the answers don’t help, get more specific from there. This is different from debugging - where you should be as specific as possible and search for exact matches. But I digress. Oh, and always prepend/append the programming/hypertext language you are referencing.
The action item is “Show a button on the page”. So let’s try see: how do we display a button in HTML? and how do I set its text? Now that we have these simple questions, we can remove some excess words and we remain with 2 great keywords to search which should get us starting on the topic, at the most general sense:
Now to answer the next burning question - how to make the button fit with the design?
We have another tricky task here.
Say our design looks something like this:
Here, if we are unfamiliar with everything, breaking down even further can really help. Let’s look at what defines the style of this button:
- It has a gradient fill/background
- It has white text
- It has rounded corners
- It has a shadow behind it
We will want to search for more specific things, which will also be our broken down tasks; such as:
Try some more simple task ideas, and practice breaking down tasks into chunks that you can definitely complete. As tasks become more complex, they will sometimes become harder. But sometimes you will find that they don’t, really — they just become longer, and larger, which may seem daunting, but all big tasks can sometimes be broken up into many, many tiny tasks, which will be easy to actually get done.
Any progress is important: No task is too small
One of the important concepts behind this approach is that tasks should be broken down until you can picture in your mind the entire task’s execution, to some extent. It’s not really a rule to follow, but more of a guiding line. Tasks that you can picture yourself doing almost fully, you can probably complete. And if the smallest task is still beyond your knowledge, then you try to break it down further, or must go out and seek that knowledge.
Which is to say - in order to make your tasks useful, you must make them doable. Once you can tick a checkbox that says “I’m done”, no matter the size of the contribution to the total pool of tasks, you still made some progress. That progress piles up slowly, and soon you will see your projects come to life.
The next steps
Practice makes perfect - so whatever side project you have, apply this new knowledge to it. Take your tasks, ask the questions, and turn them into smaller ones.
In the next part of this series, we will take a look at some more complex examples - abstract, large ideas that need to become a coded reality. For the meantime, a good grasp of simple tasks breakdown will shape your minds properly for the next difficulty that lies ahead.
About the author
My name is Chen Asraf. I’m a programmer at heart — it's both my job that I love and my favorite hobby. Professionally, I make fully fledged, production-ready web, desktop and mobile apps for start-ups and businesses; or consult, advise and help train teams.
I'm passionate about tech, problem solving and building things that people love. Find me on social media:
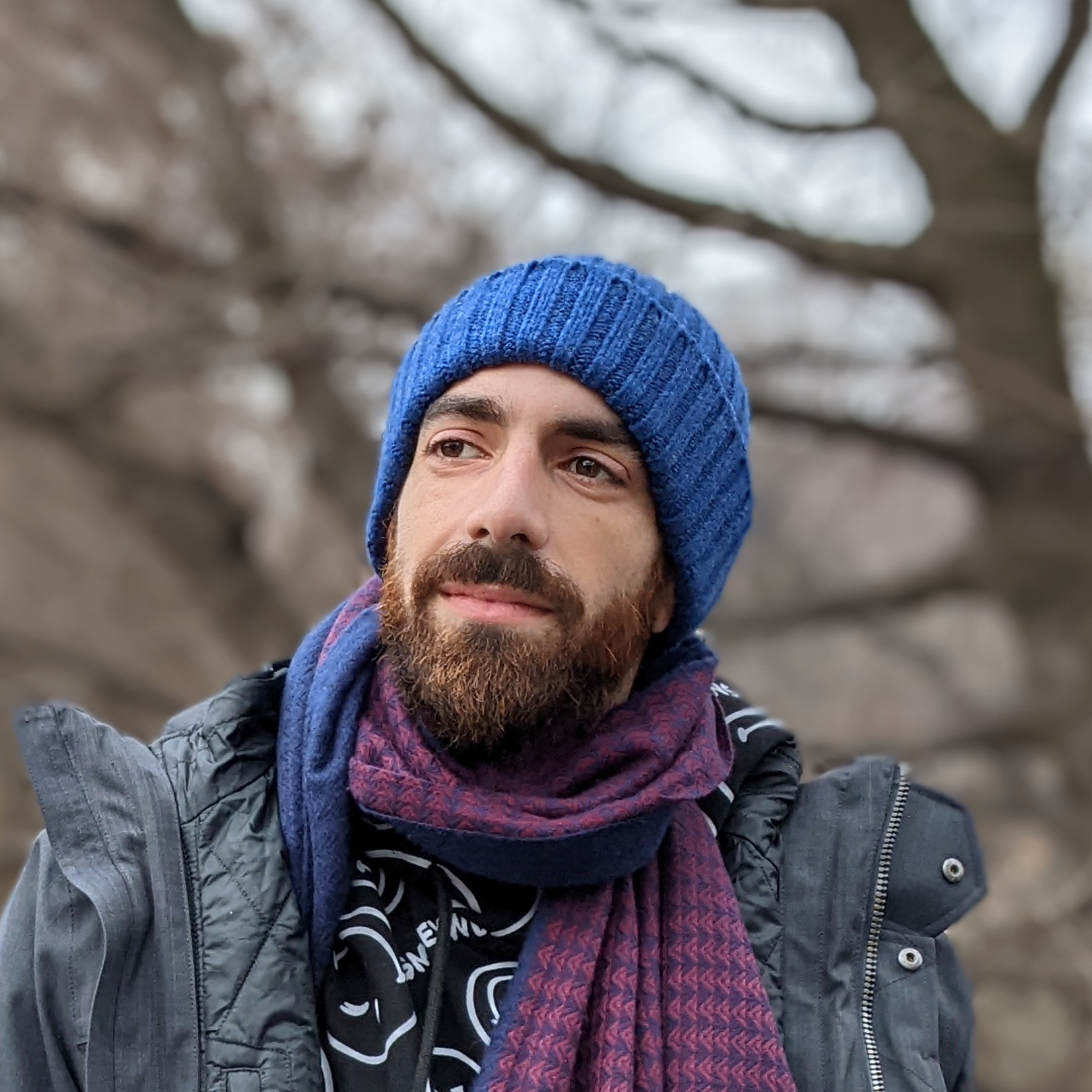